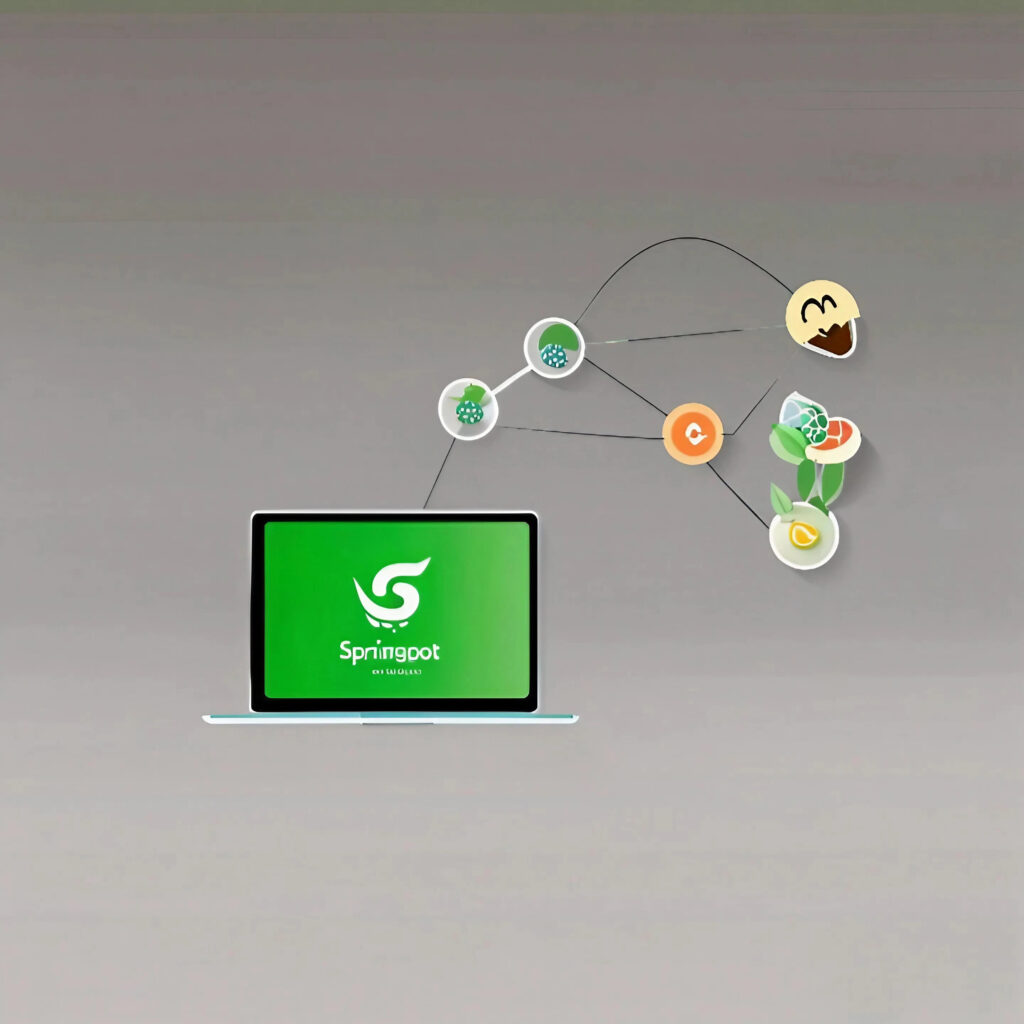
Introduction to Top 10 most common spring boot interviews questions
Introduction to Top 10 most common spring boot interviews questions
Table of Contents
Entering the world of Spring boot development can be both exciting and challenging. To help you prepare for your upcoming Spring boot interviews, we’ve compiled a list of commonly asked questions to give you a head start. Whether you’re aiming for your, understanding these concepts will showcase your knowledge and readiness to work with Spring boot. Let’s dive into the key interview questions that every professional should be familiar with.
In this blog post, we’ll provide expert answers to the top 10 spring boot questions commonly asked in interviews, helping you master spring boot. Let’s dive in!
1. How to connect to 2 data sources in springboot ?
In Spring Boot, you can connect to multiple data sources by configuring multiple DataSource
beans and then using these beans to interact with the respective databases. Here’s a step-by-step guide on how to achieve this:
Configure Data Source Properties: In your application.properties
or application.yml
file, define the properties for each data source separately. For example:
# Data Source 1 spring.datasource.url=jdbc:mysql://localhost:3306/datasource1 spring.datasource.username=username1 spring.datasource.password=password1 spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver # Data Source 2 datasource2.url=jdbc:mysql://localhost:3306/datasource2 datasource2.username=username2 datasource2.password=password2 datasource2.driver-class-name=com.mysql.cj.jdbc.Driver
- Create Configuration Classes: Create two configuration classes, each representing a data source, and use the properties defined above to configure the respective
DataSource
beans.
For example:
@Configuration @EnableTransactionManagement public class DataSource1Config { @Bean(name = "dataSource1") @ConfigurationProperties(prefix = "spring.datasource") public DataSource dataSource1() { return DataSourceBuilder.create().build(); } // Other configurations for Data Source 1, like JdbcTemplate, JpaVendorAdapter, etc. }
@Configuration @EnableTransactionManagement public class DataSource2Config { @Bean(name = "dataSource2") @ConfigurationProperties(prefix = "datasource2") public DataSource dataSource2() { return DataSourceBuilder.create().build(); } // Other configurations for Data Source 2, like JdbcTemplate, JpaVendorAdapter, etc. }
Use Multiple Data Sources in Services/Repositories: Now, you can use the data sources in your services or repositories by autowiring the respective DataSource
beans:
@Service public class MyService { private final DataSource dataSource1; private final DataSource dataSource2; @Autowired public MyService(@Qualifier("dataSource1") DataSource dataSource1, @Qualifier("dataSource2") DataSource dataSource2) { this.dataSource1 = dataSource1; this.dataSource2 = dataSource2; } // Your service logic using dataSource1 and dataSource2 }
Make sure to use the @Qualifier
annotation to specify which data source should be injected in the service constructor.
- Handle Transactions: If you want to perform transactions across multiple data sources, you need to configure a JTA (Java Transaction API) manager. Spring Boot supports JTA through third-party libraries like Atomikos or Bitronix. By integrating these libraries, you can manage distributed transactions across multiple data sources.
Remember to include the necessary dependencies for your chosen JTA library in your pom.xml
or build.gradle
file.
With these steps, you’ll be able to connect and interact with multiple data sources in your Spring Boot application.
2. How does Spring Boot handle configuration properties?
Spring Boot handles configuration properties through a combination of application.properties or application.yml files, and configuration classes annotated with @ConfigurationProperties.
You can use application.properties or application.yml to define properties that can be used to configure various aspects of your application, such as database connection details, server port, etc. These files can be placed in the classpath of your application, and are loaded by Spring Boot automatically.
You can also use the @ConfigurationProperties annotation to bind properties to Java bean classes. This allows you to define and validate configuration properties in a type-safe manner, making it easier to maintain and evolve your application. With this annotation, you can specify the properties to bind by specifying the prefix of the properties, and then you can access the properties in your code using the annotated bean.
Spring Boot also provides several ways to externalize configuration, so you can easily manage different configurations for different environments, such as development, testing, and production. For example, you can use command line arguments, system properties, or environment variables to override properties defined in the application.properties or application.yml file.
3. Can you explain how Spring Boot handles logging?
Spring Boot handles logging by automatically configuring a logging system, such as Logback, based on the dependencies present in the classpath. By default, Spring Boot uses Logback as its logging system, but you can use other logging systems such as Log4j2 if you include the required dependencies in your classpath.
When you run a Spring Boot application, Spring Boot automatically sets up the logging system and outputs log messages to the console. You can control the logging configuration, such as the logging level, through the application.properties or application.yml file. For example, you can set the logging level to debug by adding the following line to the application.properties file: logging.level.root=debug
You can also customize the logging configuration by creating a logback-spring.xml file in the classpath of your application. This file allows you to configure various aspects of the logging system, such as the logging pattern, appender, and layout.
In addition to the console output, you can also configure Spring Boot to write log messages to a file, send them to a remote server, or output them to other log destinations using appenders. This allows you to manage and analyze your log messages more effectively and to diagnose issues in your application more efficiently.
4. How does Spring Boot handle security?
Spring Boot handles security through the Spring Security framework, which provides a comprehensive security solution for Java applications. Spring Security can be configured either programmatically or through XML
configuration files. In a Spring Boot application, security can be easily enabled by including the spring-boot-starter-security dependency in the classpath.
By default, Spring Boot sets up a basic security configuration that requires users to authenticate using a user name and password. The user name and password can be configured in the application.properties or application.yml file. For example:
spring.security.user.name=user
spring.security.user.password=password
In addition to basic authentication, Spring Security also supports a wide range of authentication mechanisms, including LDAP, OAuth 2.0, and JWT. You can configure these mechanisms by writing custom authentication providers or by using pre-configured security modules.
Spring Security also provides a flexible authorization mechanism that allows you to define access control rules based on the roles and permissions of authenticated users. For example, you can restrict access to certain parts of your application based on the user’s role, such as only allowing administrators to access the administrative pages.
Spring Boot provides a number of options for customizing the security configuration, such as configuring HTTPS, setting up CSRF protection, and customizing the authentication and authorization rules. By using the Spring Security framework, you can easily secure your Spring Boot application and protect sensitive data.
5. How does Spring Boot handle data access and persistence?
Spring Boot handles data access and persistence using the following main components:
Spring Data: A set of libraries for working with different data sources, including databases, NoSQL data stores, and cloud-based data stores.
JPA (Java Persistence API): An API for accessing and managing relational databases, typically implemented by Hibernate.
Spring Data JPA: A library that provides an abstraction over JPA and makes it easier to work with relational databases in a Spring Boot application.
JDBC (Java Database Connectivity): A low-level API for accessing and managing relational databases.
Spring JDBC: A library that provides an abstraction over JDBC and makes it easier to work with relational databases in a Spring Boot application.
Transactions: A mechanism for ensuring that database operations are atomic, consistent, isolated, and durable (ACID).
Object-Relational Mapping (ORM): A technique for mapping objects in a program to records in a relational database.
In a Spring Boot application, you typically declare the dependencies you need for data access and persistence in your build file (e.g., Maven or Gradle), and then use the APIs and annotations provided by Spring Boot to access and persist data.
6. Can you explain how Spring Boot handles web development?
Spring Boot handles web development by providing a set of libraries and tools for building web applications. Some key components are:
Spring MVC: A framework for building web applications that uses the Model-View-Controller (MVC) design pattern.
Templates: Tools for rendering HTML, such as Thymeleaf or FreeMarker, that can be used to dynamically generate web pages.
Static Content: A way to serve static resources, such as images, stylesheets, and JavaScript files, directly to clients.
RESTful Web Services: A way to build web services that conform to the Representational State Transfer (REST) architectural style, using libraries such as Spring Data REST.
WebSockets: A real-time communication protocol for sending messages between a client and a server over a single, long-lived connection.
Security: A way to secure your web application, using libraries such as Spring Security.
Testing: A way to test your web application, using libraries such as Spring Boot Test or JUnit.
7. How does Spring Boot handle deployment and production-readiness?
Spring Boot handles deployment and production-readiness through a number of features, including:
Embeddable Applications: Spring Boot applications are designed to be easy to run as standalone applications, with embedded web servers such as Tomcat or Jetty. This means that they can be deployed without the need for a separate application server.
Externalized Configuration: Spring Boot applications can be configured using properties files, YAML files, command-line arguments, and other sources, which makes it easy to manage configuration for different environments (e.g., development, test, and production).
Health Indicators: Spring Boot provides a number of pre-configured health indicators that you can use to monitor the health of your application and its dependencies, such as the database and the file system.
Actuators: A set of endpoints that provide information about the health, configuration, and other aspects of a running Spring Boot application.
Metrics: A way to collect and report metrics about your application, such as the number of requests served, the response times, and the memory usage.
Logging: Spring Boot provides a flexible logging infrastructure that supports a variety of logging frameworks (e.g., Logback, Log4j) and makes it easy to configure logging for different environments.
Profiles: A way to activate different configurations based on the environment in which your application is running, such as development, test, or production.
Monitoring: A way to monitor the performance and health of your application in real-time, using tools such as JMX, Micrometer, or Actuator. Overall, Spring Boot makes it easy to build and deploy applications that are production-ready, with features such as monitoring, logging, and configuration management.
8. How does Spring Boot support unit and integration testing?
Spring Boot provides several features to support unit and integration testing in your applications.
Unit tests:
Spring Boot provides a TestRestTemplate class for testing REST endpoints.
You can use the @SpringBootTest annotation to load a full application context for your tests.
You can use the @MockBean annotation to add mock implementations of required beans to the application context.
You can use the @DataJpaTest annotation to load a test application context configured for JPA testing.
Integration tests:
Spring Boot provides the @SpringBootTest annotation to load a full application context for your tests.
You can use the @TestPropertySource annotation to specify properties to use during testing.
You can use the TestRestTemplate class to test REST endpoints.
You can use the MockMvc class to perform HTTP requests to a server and test the results, without the need to start a full HTTP server.
9. Can you explain how Spring Boot handles microservices?
Spring Boot provides several features to support the development of microservices using Spring:
Embedded servers: Spring Boot comes with embedded servers, such as Tomcat and Jetty, allowing you to run your application without the need to install and configure a separate application server.
Auto-configuration: Spring Boot provides auto-configuration for many common microservice components, such as security, caching, data access, and more. This allows you to get started quickly without having to configure everything yourself.
RESTful services: Spring Boot provides a simple and flexible way to create RESTful web services using Spring MVC. You can define your REST API using annotations and use the RestTemplate class to perform HTTP requests to your API.
Service discovery: Spring Boot provides support for service discovery using Spring Cloud and Netflix Eureka. Service discovery allows your microservices to find and communicate with each other at runtime, reducing the need for hard-coded service addresses.
Load balancing: Spring Boot provides support for load balancing using Spring Cloud and Netflix Ribbon. Load balancing allows you to distribute incoming requests across multiple instances of your microservices, improving performance and reliability.
Configuration management: Spring Boot provides support for centralized configuration management using Spring Cloud and Apache ZooKeeper. Centralized configuration management allows you to manage configuration properties for your microservices in a single place, reducing the need for manual configuration changes.
10. How do you use Spring Boot to create a CLI (Command Line Interface) application?
Create a Spring Boot project using the Spring Initializer or your preferred IDE.
Add the Spring Boot CLI dependency to your project’s pom.xml file:
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-cli</artifactId> <version>2.3.3.RELEASE</version> </dependency
Create a Groovy script that defines your CLI command, annotate it with @CommandLineRunner, and specify its behavior in the run method. For example
import org.springframework.boot.CommandLineRunner import org.springframework.stereotype.Component @Component class HelloWorld implements CommandLineRunner { @Override void run(String... args) { println("Hello World!") } }
Run your script using the spring run command and passing your script file as an argument.
spring run hello-world.groovy
Optionally, you can also package your script as a standalone jar by running ./mvnw package and executing the resulting jar file using java -jar my-cli.jar.
Conclusion:
By understanding and practicing these top 10 springboot interview questions, you can showcase your proficiency in springboot and increase your chances of success in interviews. Remember to explore additional resources, continue coding exercises, and stay updated with the latest trends in Spring boot . Best of luck on your Python interview journey!
If you found this blog post helpful, make sure to check out our other blog posts on JavaScript, HTML, CSS and other programming languages to expand your knowledge and excel in your programming journey.
Remember, continuous learning and practical implementation are key to becoming a proficient Spring Boot. Good luck with your SpringBoot interviews and happy coding!