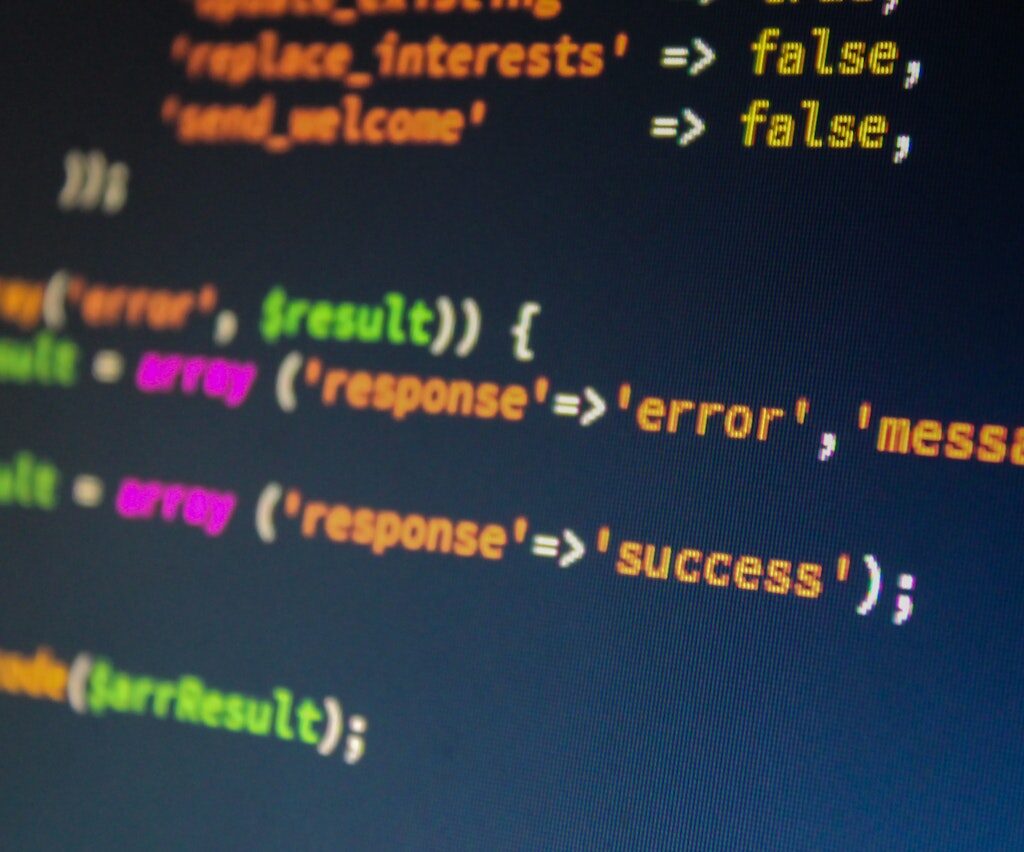
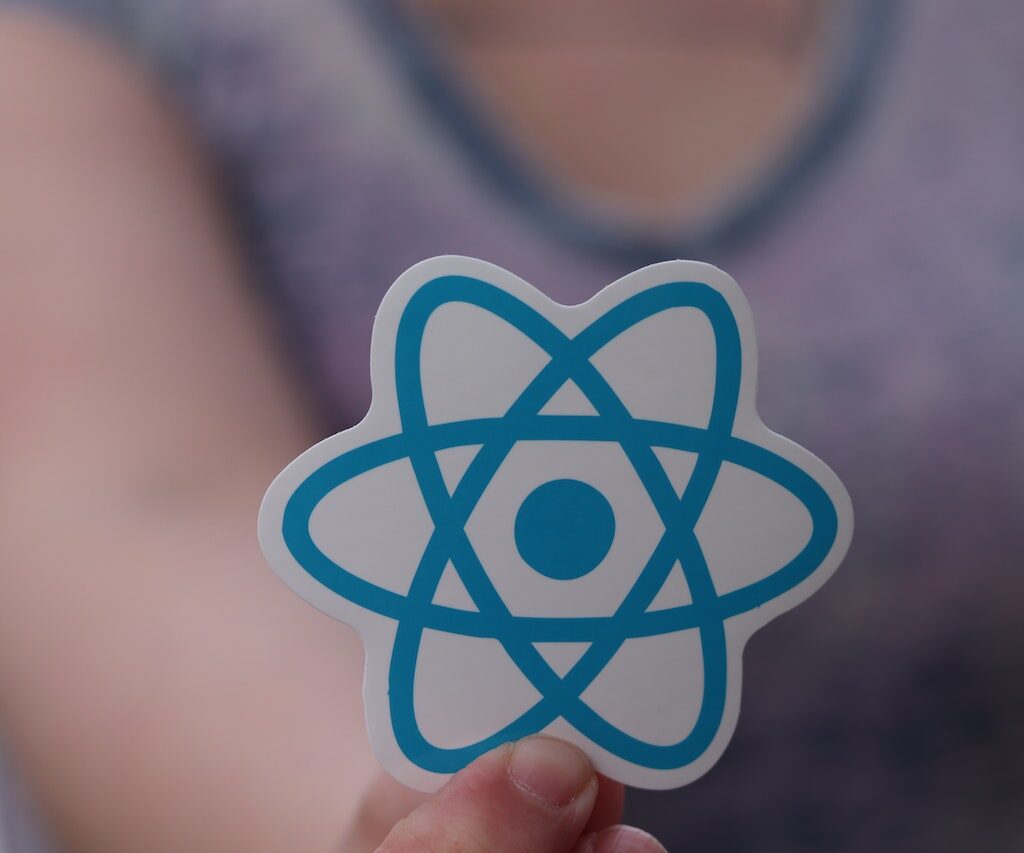
Introduction to Top 10 most common react for fresher level interviews questions
Introduction to Top 10 most common React for fresher level interviews questions
Table of Contents
Entering the world of React development as a fresher can be both exciting and challenging. To help you prepare for your upcoming React interviews, we’ve compiled a list of commonly asked questions to give you a head start. Whether you’re aiming for your first React job or an internship, understanding these concepts will showcase your knowledge and readiness to work with React. Let’s dive into the key interview questions that every fresher should be familiar with.
In this blog post, we’ll provide expert answers to the top 10 React questions commonly asked in interviews, helping you master python and excel in fresher experience level interviews. Let’s dive in!
1. What is React? How does it differ from other JavaScript frameworks?t?
React is a widely-used JavaScript library for building user interfaces. It stands out for its virtual DOM, which efficiently updates and renders components by calculating the minimal changes needed. React’s component-based architecture promotes code reusability and maintainability, allowing developers to create modular UIs. With JSX, developers can write HTML-like code within JavaScript, making it easier to describe the structure and layout of components. Unlike full-fledged frameworks, React focuses mainly on the view layer, offering flexibility to integrate with other tools for routing, state management, and more. Its popularity has fostered a vast ecosystem of libraries and resources, providing solutions for various needs. This thriving community support, combined with React’s performance and flexibility, makes it an appealing choice for building modern web applications.
In summary, React is a JavaScript library known for its virtual DOM, component-based architecture, and JSX syntax. It offers a focused approach to building UIs and can be complemented with other tools for a comprehensive application development. React’s ecosystem provides extensive resources and community support, making it a popular choice for developers aiming to create dynamic and efficient user interfaces.
2. Explain the concept of JSX in React?
JSX is an extension to the JavaScript syntax used in React. It allows developers to write HTML-like code within JavaScript, simplifying the creation of UI components. Here’s an example of JSX code:
const element = <h1>Hello, JSX!</h1>;
In the above code, the JSX expression <h1>Hello, JSX!</h1>
represents a React element that renders a heading with the text “Hello, JSX!”. This JSX code will be transformed into equivalent JavaScript code by the React compiler before it is executed.
3. What are the key features of React?
- Virtual DOM: React uses a virtual DOM, which is a lightweight representation of the actual browser DOM. It allows React to efficiently update and render components by calculating and applying only the necessary changes. This approach improves performance and ensures a smooth user experience.
- Component-Based Architecture: React follows a component-based approach, where UIs are divided into reusable and self-contained components. Each component manages its own state, allowing for easier maintenance, reusability, and composability. Components can be combined to build complex UIs.
- JSX: JSX is an extension to JavaScript syntax that allows developers to write HTML-like code within JavaScript. JSX makes it easier to describe the structure and layout of components, providing a more intuitive way to define the UI.
- Unidirectional Data Flow: React enforces a unidirectional data flow, which means data flows in a single direction within the component hierarchy. This makes it easier to understand and debug the application’s state changes, leading to more predictable and manageable code.
- Developer Tools: React comes with a set of powerful developer tools that help with debugging, inspecting component hierarchies, and monitoring performance. These tools make the development process more efficient and enable developers to build high-quality applications.
- Large Ecosystem: React has a vast and active community, resulting in a rich ecosystem of libraries, tools, and resources. This ecosystem provides solutions for various needs, including state management, routing, testing, styling, and more. Developers can leverage this ecosystem to enhance and extend their React applications.
Overall, React’s key features such as virtual DOM, component-based architecture, JSX, unidirectional data flow, developer tools, and a thriving ecosystem contribute to its popularity and make it a powerful choice for building modern web applications.
4. What is the virtual DOM in React? How does it improve performance?
The virtual DOM is a key concept in React that is used to efficiently update and render components. It is a lightweight representation of the actual browser DOM (Document Object Model).
Here’s how it works:
- When a component’s state changes in React, a new virtual DOM tree is created, representing the updated UI based on the new state.
- React then performs a diffing algorithm to calculate the differences between the previous virtual DOM tree and the new one.
- Once the differences are identified, React updates only the necessary parts of the actual browser DOM, rather than re-rendering the entire page.
This approach offers several performance benefits:
- Efficient Updates: By comparing the virtual DOM trees and updating only the necessary changes in the actual DOM, React minimizes the amount of work needed during the update process. This leads to faster and more efficient updates, particularly when dealing with complex UIs.
- Batched Updates: React also batches multiple updates together, reducing the number of DOM operations and optimizing performance. This helps prevent unnecessary reflows and repaints in the browser, resulting in smoother rendering.
- Virtual DOM as an In-Memory Representation: The virtual DOM operates in memory and is faster to manipulate compared to the actual browser DOM. This allows React to perform calculations and updates more efficiently, as it avoids the performance overhead associated with directly modifying the browser DOM.
By leveraging the virtual DOM, React abstracts away the complexities of directly manipulating the browser DOM and provides a more efficient approach to update and render components. It helps ensure a responsive and smooth user experience, especially when dealing with dynamic and interactive UIs.
5. What are React components? Describe the difference between functional and class components?
In React, components are the building blocks of user interfaces. They encapsulate the logic and rendering of a part of the UI, making it reusable and modular. Components can be thought of as self-contained, independent units that can be combined to create complex UI structures.
There are two main types of components in React: functional components and class components.
- Functional Components:
- Functional components are defined as JavaScript functions.
- They receive props (input data) as arguments and return JSX elements that represent the UI.
- Functional components are simple and straightforward, focusing solely on rendering based on the input data.
- They are preferred when the component doesn’t need to manage its own state or lifecycle methods.
- Functional components are easier to read, test, and optimize for performance.
- Since React introduced hooks, functional components can also have their own local state and lifecycle management using hooks.
Here’s an example of a functional component:
function Greeting(props) {
return <h1>Hello, {props.name}!</h1>;
}
- Class Components:
- Class components are defined as JavaScript classes that extend the
React.Component
class. - They have more features and capabilities compared to functional components.
- Class components can manage their own state, handle lifecycle methods (such as
componentDidMount
andcomponentDidUpdate
), and handle complex logic. - They are suitable for components that require more advanced functionality and have more complex state management needs.
- However, class components tend to have more boilerplate code and can be harder to read and understand.
- Class components are defined as JavaScript classes that extend the
Here’s an example of a class component:
class Greeting extends React.Component {
render() {
return <h1>Hello, {this.props.name}!</h1>;
}
}
With the introduction of React Hooks, functional components have become the preferred approach in React development due to their simplicity and ease of use. However, class components are still used in legacy codebases or when working with libraries that rely on class-based components.
It’s worth noting that with React 17 and later versions, functional components and class components have more parity in terms of features, as functional components can handle state and lifecycle management through hooks.
6. What is the purpose of state in React? How is it different from props?
Consider a Counter
component in React:
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
const incrementCount = () => {
setCount(count + 1);
};
return (
<div>
<p>Count: {count}</p>
<button onClick={incrementCount}>Increment</button>
</div>
);
}
export default Counter;
In this example, we have a functional component called Counter
. It uses the useState
hook, a React feature for managing state within functional components.
The count
state variable is initialized with a default value of 0 using useState(0)
. It represents the current count value, which can change over time. The setCount
function is used to update the state and trigger a re-render of the component.
When the Increment
button is clicked, the incrementCount
function is called, which increases the count
value by 1 using setCount(count + 1)
. This triggers a re-render, updating the UI to display the new count value.
Now, let’s consider a parent component that uses the Counter
component:
import React from 'react';
import Counter from './Counter';
function App() {
return <Counter />;
}
export default App;
In this example, the App
component renders the Counter
component. Here, the Counter
component does not receive any props from its parent. It manages its own state internally using the useState
hook.
The key difference between state and props is that state is managed within the component itself, allowing it to hold and update data that affects its behavior and rendering. On the other hand, props are passed from parent components and provide a way to configure and pass data to child components.
In summary, state is used to manage internal data within a component, allowing it to hold and update information that affects its behavior and rendering. Props, on the other hand, are used for passing data from parent components to child components, enabling configuration and customization of the child components.
7. How do you handle events in React? Provide an example?
In React, handling events involves attaching event handlers to elements within components. Event handlers are functions that are executed when a specific event occurs, such as a button click or form submission. React provides a syntax for defining event handlers and binding them to elements.
Here’s an example of how to handle an onClick event in React:
import React from 'react';
function Button() {
const handleClick = () => {
console.log('Button clicked!');
};
return (
<button onClick={handleClick}>
Click Me
</button>
);
}
export default Button;
In the above example, we have a functional component called Button
that renders a button element. The handleClick
function is the event handler for the onClick
event of the button.
When the button is clicked, the handleClick
function is executed. In this example, it simply logs a message to the console.
The handleClick
function is bound to the button’s onClick
event using the onClick
attribute. Note that we pass the function reference handleClick
rather than invoking it directly with parentheses.
By using this syntax, React takes care of event delegation and ensuring the event handlers are executed correctly.
This is just a basic example, but event handling in React can also involve passing data, updating state, or triggering other actions based on the event. Event handling in React follows a similar pattern for various events such as onChange, onSubmit, onMouseOver, etc.
8. What is the significance of keys in React lists?
Keys in React lists are special attributes that provide a unique identifier to each item in the list. They enable efficient updating and reconciliation of dynamic lists, ensuring stable component instances and preventing unnecessary re-renders. Keys play a crucial role in optimizing performance when working with lists of elements in React.
9. Explain the React component lifecycle methods?
- React component lifecycle methods are functions invoked at specific stages of a component’s lifespan.
- Mounting phase methods include
constructor
for initialization,render
for creating the UI, andcomponentDidMount
for post-render tasks. - Updating phase involves re-rendering with
render
and usingcomponentDidUpdate
for post-update operations. - Unmounting phase executes
componentWillUnmount
just before a component is removed from the DOM. - React Hooks provide an alternative to lifecycle methods for functional components, with
useEffect
being a popular hook for handling side effects and state management. - Some lifecycle methods have been deprecated, and developers should refer to the React documentation for version-specific information and best practices.
10. How do you pass data between parent and child components in React?
In React, data can be passed from parent components to child components using props. Props (short for properties) are a way to pass data and configuration from a parent component to its child components.
Here’s an example of how data is passed from a parent component to a child component:
Parent component:
import React from 'react';
import ChildComponent from './ChildComponent';
function ParentComponent() {
const data = 'Hello from Parent';
return (
<div>
<ChildComponent message={data} />
</div>
);
}
export default ParentComponent;
Child component:
import React from 'react';
function ChildComponent(props) {
return (
<div>
<p>{props.message}</p>
</div>
);
}
export default ChildComponent;
In this example, the ParentComponent
renders the ChildComponent
and passes the data
variable as a prop named message
. The child component receives this prop using the props
object and can access it as props.message
.
By passing props from the parent to the child component, the child component can use the received data to render content, make decisions, or perform other logic. Props are read-only and cannot be modified by the child component.
Parent components can pass any type of data as props, including strings, numbers, booleans, objects, or even functions. This allows for flexible and dynamic communication between components in a React application.
In summary, data is passed from parent to child components in React through props. Parent components provide data by specifying props when rendering child components, and child components receive and utilize the passed data through the props
object.
Conclusion:
By understanding and practicing these top 10 React interview questions for freshers, you can showcase your proficiency in React and increase your chances of success in interviews. Remember to explore additional resources, continue coding exercises, and stay updated with the latest trends in React. Best of luck on your Python interview journey!
If you found this blog post helpful, make sure to check out our other blog posts on JavaScript, HTML, CSS and other programming languages to expand your knowledge and excel in your programming journey.
Remember, continuous learning and practical implementation are key to becoming a proficient web developer. Good luck with your Web-Development fresher interviews and happy coding!