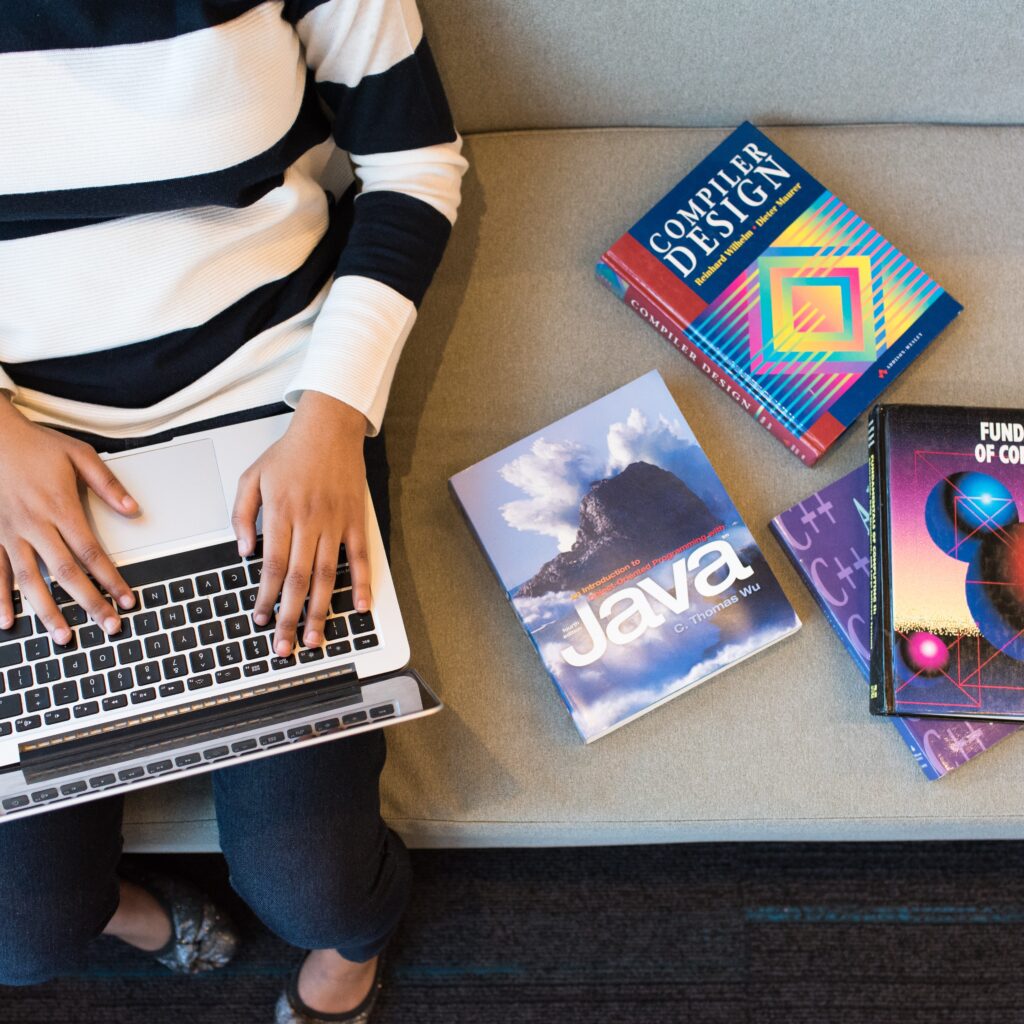
In Java, the volatile and transient keywords modify the behavior of variables. Although they may seem similar, they have distinct purposes and effects on variable handling. This article explains the difference between volatile and transient with real-world examples and code snippets.
Table of Contents
Understanding volatile IN java:
The volatile keyword is used to indicate that a variable may be modified by multiple threads and that its value should always be read from and written to the main memory, rather than cached thread-local copies. This ensures that the most up-to-date value is always accessed, even in a multi-threaded environment.
Real-world example:
Consider a scenario where multiple threads are accessing and modifying a shared boolean variable named stopFlag. The volatile keyword can be used to ensure that all threads see the latest value of stopFlag, regardless of local caching.
public class VolatileExample { private volatile boolean stopFlag = false; public void stop() { stopFlag = true; } public void run() { while (!stopFlag) { // Perform some tasks } System.out.println("Thread stopped."); } }
Understanding transient:
The transient keyword is used to indicate that a variable should not be serialized when an object is transformed into a byte stream, typically for storage or transmission purposes. It allows developers to exclude specific fields from the serialization process.
Real-world example:
Consider a class named Person that contains sensitive information, such as a social security number, which should not be serialized. By marking the ssn field as transient, we can exclude it from the serialization process.
import java.io.Serializable; public class Person implements Serializable { private String name; private transient String ssn; // Exclude from serialization public Person(String name, String ssn) { this.name = name; this.ssn = ssn; } // Getters and setters }
Conclusion:
In conclusion, we understand that
- Volatile ensures that variable changes are immediately visible to all threads.
- Transient excludes a variable from the serialization process.
References:
Java Transient
For More topics on Java:
Click Me